Getting Started with the ChatGPT API and Javascript
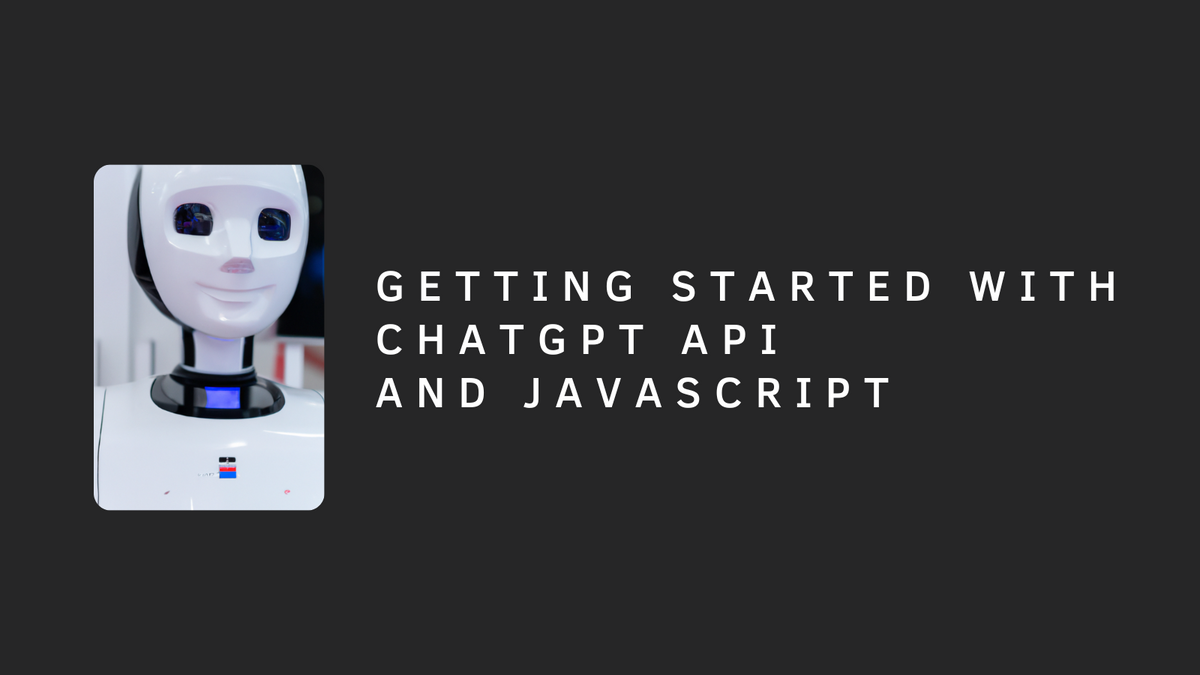
The ChatGPT API makes it super easy to create chatbots with Javascript and Node. Here's an implementation of a CLI chatbot powered by
const { Configuration, OpenAIApi } = require("openai");
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
var rl = require('readline-sync');
async function startAssistant() {
var messages = [];
var input = rl.question('What type of chatbot would you like to create? > ');
messages.push({"role": "system", "content": "Stay in character. You are " + input});
input = rl.question("Say hello to your new chatbot > ");
while(true) {
messages.push({"role": "user", "content": input});
console.log("...");
const completion = await openai.createChatCompletion({
model: "gpt-3.5-turbo",
messages: messages,
});
var reply = completion.data.choices[0].message.content;
messages.push({"role": "assistant", "content": reply});
console.log(reply);
input = rl.question("> ");
}
}
startAssistant();
And here’s what it looks like in action:
0:00
/
If you want to run this code yourself there are three key things you’ll need to do:
- Put the above code in a file code
index.js
- Use these instructions from OpenAI to save your OpenAI API key as an environment variable.
- Install these 2 dependencies:
npm install openai
npm install readline-sync
Then you can run node index.js
to interact with ChatGPT yourself.
Have fun!