Getting Started with GPT-4 API and JavaScript
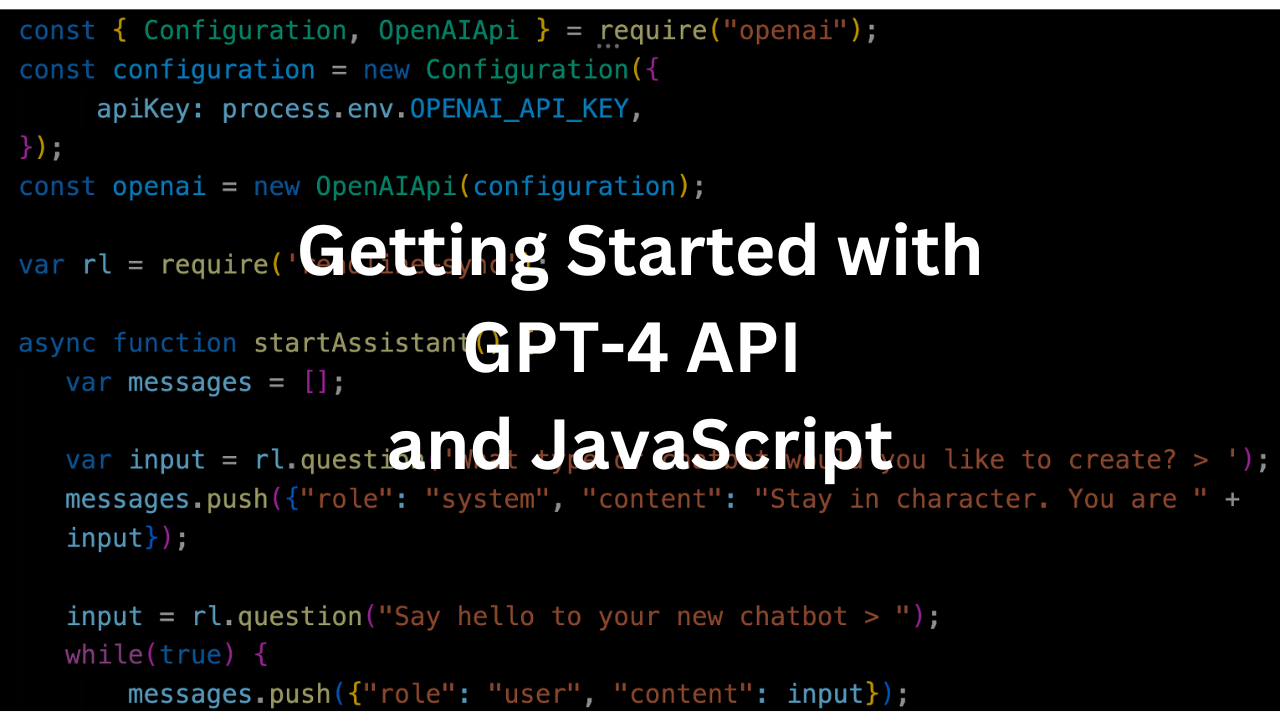
GPT-4 launched this week!
There's a waitlist for GPT-4 API access, but invites have already started going out. Once you get access, it's dead simple to get started building.
Here's a command line chatbot powered by node.js and the GPT-4 API. The only difference between this code and a Javascript bot powered by ChatGPT is that we've changed the model
parameter to gpt-4
.
const { Configuration, OpenAIApi } = require("openai");
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
var rl = require('readline-sync');
async function startAssistant() {
var messages = [];
var input = rl.question('What type of chatbot would you like to create? > ');
messages.push({"role": "system", "content": "Stay in character. You are " + input});
input = rl.question("Say hello to your new chatbot > ");
while(true) {
messages.push({"role": "user", "content": input});
console.log("...");
const completion = await openai.createChatCompletion({
model: "gpt-4",
messages: messages,
});
var reply = completion.data.choices[0].message.content;
messages.push({"role": "assistant", "content": reply});
console.log(reply);
input = rl.question("> ");
}
}
startAssistant();
Here's what that code looks like in action:
If you want to run this code yourself there are three key things you’ll need to do:
- Put the above code in a file code
index.js
- Use these instructions from OpenAI to save your OpenAI API key as an environment variable.
- Install these 2 dependencies:
npm install openai
npm install readline-sync
Then you can run node index.js
to interact with GPT-4 from the command line!
Have fun!