Map
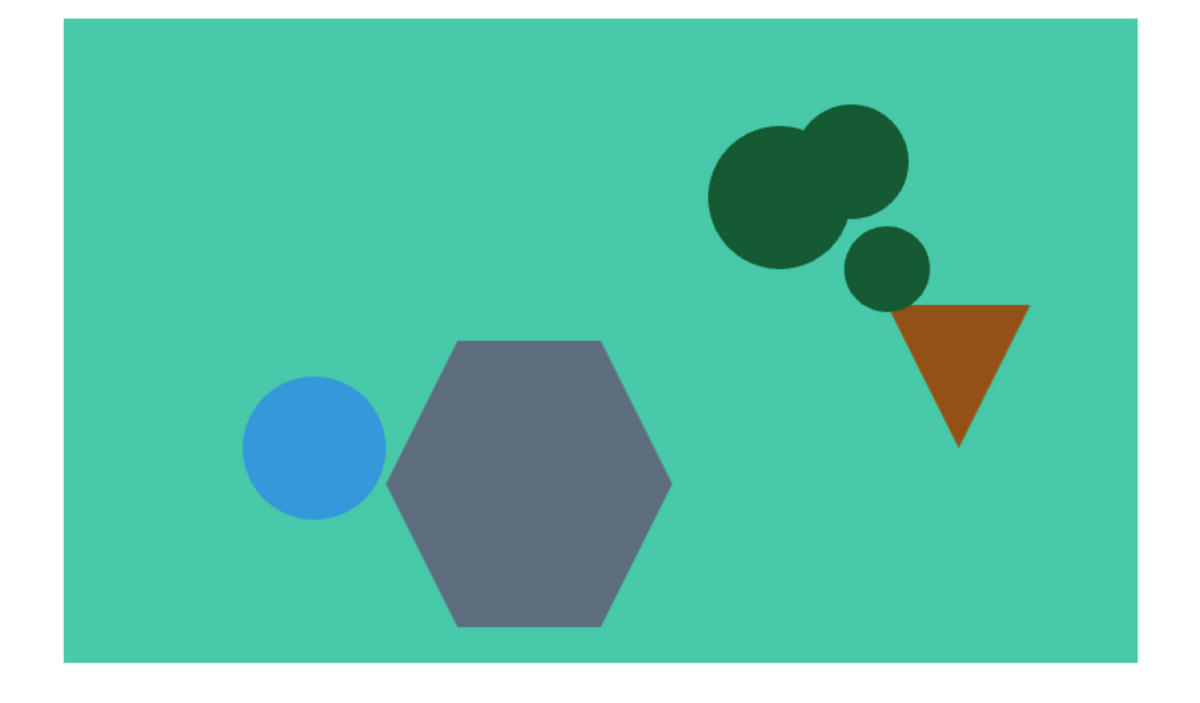
My eight year old daughter, Emma, has been writing a book called Fantasy Land about two sisters, Lillis and Neeya, who go to sleep and wake up in a world where magic is dying, and they have to save it. My best use of the ChatGPT API so far is telling it to pretend that it's Lillis, and watching Emma spend hours typing and going on adventures with a character she invented.
Last night, I read a paper by Microsoft Research which argues that GPT-4 is a significant step forward from ChatGPT – which was already blowing my mind. One example they give to illustrative the step-function improvement is asking ChatGPT (3.5 turbo) and GPT-4 to write JavaScript to produce an image similar to an example image.
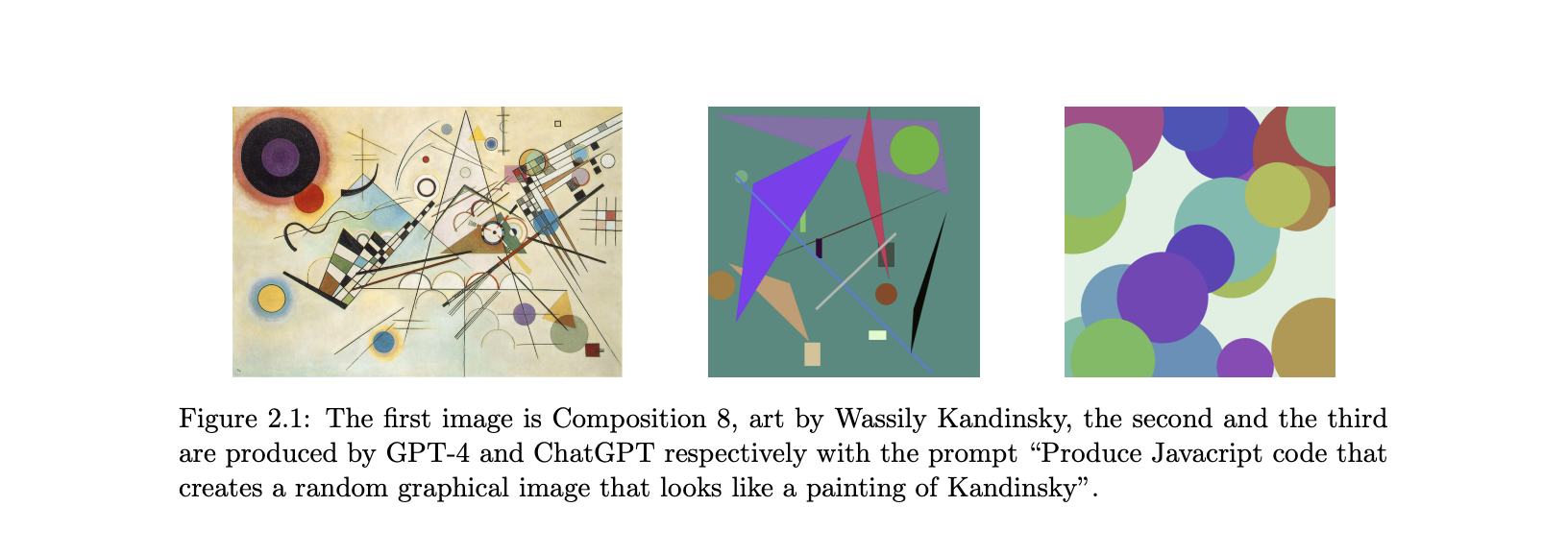
Here's the full paper: Sparks of Artificial General Intelligence: Early experiments with GPT-4.
I skimmed over this experiment at first, because my brain lumped it into the same category as what DALL-E, Midjourney, and others have been doing – creating imitations of art – which somehow, due to the pace at which everything is moving, feels old hat now. But then I re-read and realized that it wasn't just creating an image – it was writing code to create an image. There's so many layers of understanding and creation happening here.
And so, Emma and I spun up GPT-4 on the command line and gave it this prompt:
Can you please write javascript, css, and html that will draw a map for a childrens adventure video game called "fantasy land"
And here was GPT-4's reply:
Sure, let's create a simple web page with a map for a children's adventure video game called "Fantasy Land". Please note that the map is minimal and purely graphical without interactivity. You can later enhance it with more details or interactive elements per your requirements.
Here's a basic HTML, CSS, and JavaScript structure.
`index.html`
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Fantasy Land Map</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<main>
<h1>Fantasy Land Map</h1>
<canvas id="mapCanvas" width="800" height="500"></canvas>
</main>
<script src="script.js"></script>
</body>
</html>
```
`styles.css`
```css
body {
font-family: Arial, sans-serif;
background-color: #fafafa;
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
margin: 0;
padding: 0;
}
main {
background-color: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 5px 10px rgba(0, 0, 0, 0.2);
text-align: center;
}
h1 {
color: #4c4c4d;
font-size: 32px;
margin-bottom: 30px;
}
```
`script.js`
```javascript
const canvas = document.getElementById('mapCanvas');
const ctx = canvas.getContext('2d');
// Draw map background
ctx.fillStyle = '#48C9A9';
ctx.fillRect(25, 25, 750, 450);
// Draw castle
ctx.beginPath();
ctx.moveTo(400, 250);
ctx.lineTo(300, 250);
ctx.lineTo(250, 350);
ctx.lineTo(300, 450);
ctx.lineTo(400, 450);
ctx.lineTo(450, 350);
ctx.closePath();
ctx.fillStyle = '#5D6D7E';
ctx.fill();
// Draw mountain
ctx.beginPath();
ctx.moveTo(650, 325);
ctx.lineTo(600, 225);
ctx.lineTo(700, 225);
ctx.closePath();
ctx.fillStyle = '#935116';
ctx.fill();
// Draw lake
ctx.beginPath();
ctx.arc(200, 325, 50, 0, 2 * Math.PI);
ctx.fillStyle = '#3498DB';
ctx.fill();
// Draw forest
ctx.beginPath();
ctx.arc(525, 150, 50, 0, 2 * Math.PI);
ctx.fillStyle = '#145A32';
ctx.fill();
ctx.beginPath();
ctx.arc(575, 125, 40, 0, 2 * Math.PI);
ctx.fill();
ctx.beginPath();
ctx.arc(600, 200, 30, 0, 2 * Math.PI);
ctx.fill();
```
This example creates a simple map with a castle, mountain, lake, and forest. Each element is drawn using shapes such as rectangles, lines, and arcs. You can adjust the code to add or modify the elements, their colors, and positioning.
To run the project, create the 3 files `index.html`, `styles.css`, and `script.js` in the same folder, and open `index.html` in your browser.
And when I copy-pasted the reply into the three files and opened index.html
in the browser, this is what I saw:
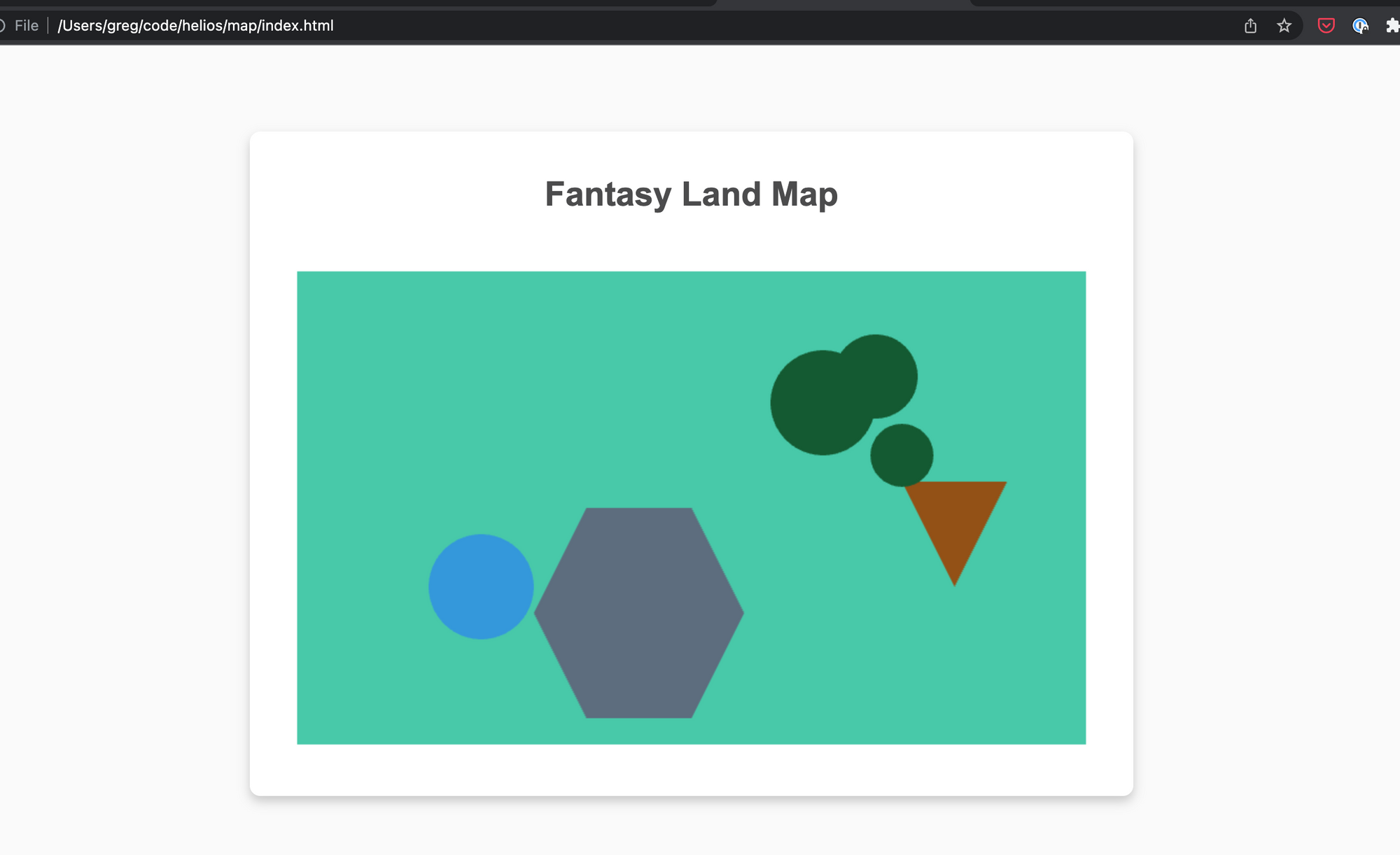
When that happened, I had to get up from my keyboard and walk around a bit to give my brain a moment to readjust its model of how the world works. Emma asked, "What's wrong Dad?" and I told her, "I've been coding for 35 years, and I can't believe that just happened."
To be clear, this doesn't even scratch the surface of what GPT-4 is capable of. This is Hello World.